Introduction
Learn how our systems securely manage API access using the OAuth 2.0 framework, ensuring authorized clients can seamlessly interact with protected resources.
API Authorization (OAuth 2.0)
Our system uses the OAuth 2.0 framework to handle secure authorization between client applications and resource servers (APIs). This ensures that only authorized clients can access our protected resources. All client applications interacting with our APIs must follow the OAuth 2.0 standard.
The OAuth 2.0 authorization framework enables a third-party application to obtain limited access to an HTTP service, either on behalf of a resource owner by orchestrating an approval interaction between the resource owner and the HTTP service, or by allowing the third-party application to obtain access on its own behalf.
OAuth defines four roles:
- Resource owner
An entity capable of granting access to a protected resource. When the resource owner is a person, it is referred to as an end-user. This is the Slade360 ecosystem user. - Resource server
The server hosting the protected resources, capable of accepting and responding to protected resource requests using access tokens. This is the can be the eTIMS server. - Client
An application making protected resource requests on behalf of the resource owner and with its authorization. The term "client" does not imply any particular implementation characteristics (e.g.,whether the application executes on a server, a desktop, or other devices). This is your application or program or piece of software you are using. - Authorization server
The server issuing access tokens to the client after successfully authenticating the resource owner and obtaining authorization. This is a Savannah Informatics Limited internal authorization server.
Our Authentication Protocol Flow
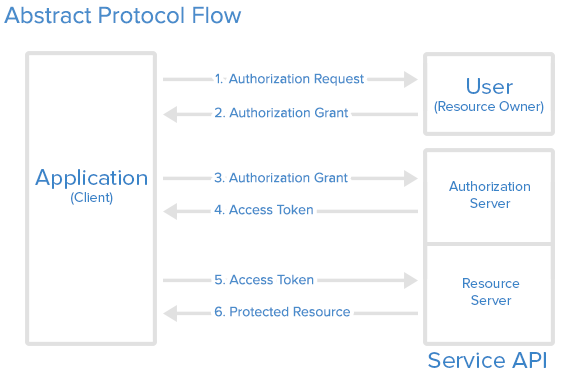
The diagram above shows the OAuth 2.0 flow, explaining how the four roles interact in these steps:
- The client requests authorization from the resource owner. The authorization request can be made directly to the resource owner (as shown), or preferably indirectly via the authorization server as an intermediary.
- The client receives an authorization grant, which is a credential representing the resource owner's authorization, expressed using one of four grant types or using an extension grant type.
The authorization grant type depends on the method used by the client to request authorization and the types supported by the authorization server. They are:- Authorization code
The authorization code is obtained by using an authorization server as an intermediary between the client and resource owner. Instead of requesting authorization directly from the resource owner, the client directs the resource owner to an authorization server, which in turn directs the resource owner back to the client with the authorization code. Here, the resource owner's credentials are never shared with the client. - Implicit
The implicit grant is a simplified authorization code flow optimized for clients implemented in a browser using a scripting language such as JavaScript. In the implicit flow, instead of issuing the client an authorization code, the client is issued an access token directly - Resource owner password credentials
The resource owner password credentials (i.e., username and password) can be used directly as an authorization grant to obtain an access token. - Client credentials
The client credentials (or other forms of client authentication) can be used as an authorization grant when the authorization scope is limited to the protected resources under the control of the client, or to protected resources previously arranged with the authorization server.
- Authorization code
- The client requests an access token by authenticating with the authorization server and presenting the authorization grant.
- The authorization server authenticates the client and validates the authorization grant, and if valid, issues an access token.
- The client requests the protected resource from the resource server and authenticates by presenting the access token.
- The resource server validates the access token, and if valid, serves the request.
The following parameters are required during the authentication protocol execution:
- Username
The username represents the user’s unique identifier in the system 1. (often an email address or user ID). It is provided by the user to authenticate their identity.- Usage
Typically used in Resource Owner Password Credentials grant types, where the user's credentials are directly exchanged for an access token. - Security Note
The username is sensitive information and must be handled securely to avoid leaks.
- Usage
- Password
The password is the secret credential tied to the username that verifies the user’s identity.- Usage
Also specific to Resource Owner Password Credentials grant types, where the password is submitted to authenticate the user. - Security Note
Avoid exposing the password in transit; always transmit it over secure protocols (e.g., HTTPS).
- Usage
- Grant Type
The grant type defines the type of authorization flow used in the OAuth 2.0 process. Our authentication server uses this grant type:- Resource Owner Password Credentials
Direct exchange of username and password for an access token. - Usage
Defines the workflow for obtaining an access token. - Security Note
We picked this appropriate grant type for the use case to ensure secure token issuance.
- Resource Owner Password Credentials
- Client ID
The client ID is a public identifier for the client application. It helps the authorization server recognize which application is making the request.- Usage
Sent with every request during the authentication process. - Security Note
While not a secret, we ensure the client ID is not easily guessable to prevent misuse.
- Usage
- Client Secret
The client secret is a confidential key issued to the client application during registration. It is used to authenticate the client with the authorization server.- Usage
Typically combined with the client ID to form a credential pair. Required in grant types like Client Credentials or Authorization Code. - Security Note
We never expose the client secret in the client-side code or publicly accessible areas. Always store it securely (e.g., in environment variables or secure storage).
- Usage
Click here for detailed information on OAuth 2.0
How It Works
Before initiating the protocol, the client registers with the authorization server. This is an internal process (Contact us for this step). Our client registration does not require a direct interaction between the client and the authorization server.
Our registration rely on redirection a URI (Uniform Resource Identifier), and client type.
When registering a client, we SHALL:
- Specify the client type
- Provide its client redirection URIs
- Application name
- Website
- Description
- Acceptance legal term
Client Types
We defines two client types based on their ability to authenticate securely with our authorization server.
- Private
Clients capable of maintaining the confidentiality of their credentials, or capable of secure client authentication using other means. This are majorly our internal services. - Public
Clients incapable of maintaining the confidentiality of their credentials, and incapable of secure client authentication via any other means. This is what our Slade360 ecosystem APIs fall.
Our authorization server DOES NOT make assumptions about the client type.
Client Identifier
Our authorization server issues the registered client a client identifier a unique string representing the registration information provided by the client.
The client identifier is not a secret. It is exposed to the resource owner and IS NOT used alone for client authentication. The client identifier is unique to our authorization server.
Client Authentication
If the client type is private, the client and our authorization server establish a client authentication method suitable for the security requirements of the authorization server.
Our authorization server accepts a specific form of client authentication meeting our security requirements.
Our private clients are typically issued a set of client credentials used for authenticating with our authorization server i.e password
Our authentication scheme is based on the model that the client must authenticate itself with a user-ID and a password for each realm. The realm value should be considered an opaque string which can only be compared for equality with other realms on that server.
The authenticating server will service the request only if it can validate the user-ID and password for the protection space of the Request-URI.
Client Password
Clients in possession of a client password use the HTTP Basic authentication scheme to authenticate with our authorization server.
We encode the client identifier using the "application/x-www-form-urlencoded" encoding algorithm, and the encoded value is used as the username, the client password is encoded using the same algorithm and used as the password.
Our authorization server supports including the client credentials in the request-body using the following parameters:
- client_id
This is a REQUIRED attribute. This is the client identifier issued to the client during the registration process. It helps to identify the target resource. - client_secret
This is a REQUIRED attribute. The client secret is issued to the client during the registration process.
Our authorization server requires the use of TLS(Transport Layer Security), when sending requests using password authentication.
Since our client authentication method involves a password, our authorization server protects any endpoint utilizing it against brute force attacks.
Client Requests Authorization
Before accessing Slade360 ecosystem APIs, the client (a web app, mobile app, or server) must request an access token from our authorization server.
Authorization Server Grants Token
The Slade360 ecosystem authorization server will generate, and return an access token if the client provides valid credentials(like a client ID and secret).
Client Uses Token to Access Slade360 Ecosystem APIs
The client (a web app, mobile app, or server) MUST include this access token in the Authorization header of every API request. The resource server (API) checks the token's validity. If the token is valid, the server grants access to our requested resource.
Example
Let's consider an application that needs to access customer records from a secure application API. Here's how it would follow the OAuth 2.0 process in our secure ecosystem:
-
Request an Access Token
The application (client) first requests an access token from our internal authorization server to securely access the customer records.
POST /oauth2/token/ Host: https://accounts.multitenant.slade360.co.ke/ Content-Type: application/x-www-form-urlencoded
Authentication Parameters Authorization Values username Email for the user password Password for the user grant_type Password for the user client_id Client ID generated by authentication server client_secret Client secret generated by authentication server
-
Our Authorization Server Response
Our authorization server, upon validating the credentials, will respond with an access token and a refresh token.
The refresh_token allows the client to request a new access_token once the current one expires, without the need for re-authentication.
{ "access_token": "xyz789secureToken", "token_type": "Bearer", "expires_in": 3600, "scope": "advantage.appointment.read advantage.appointment.write", "refresh_token": "abc123refreshToken" }
-
Use the Access Token to Request Customer Data
The application then includes this token in the header of the API request to access customer data.
curl --request GET \ --url http://accounts.core.slade360.co.ke/v1/user/me/ \ --header 'Authorization: bearer RnKpQzM2oSQ69kQXzWiadDHb2SB4fE'
Example
GET /api/customers/12345/records Host: medical-api.com Authorization: Bearer xyz789secureToken
-
API Server Responds with Data
If the token is valid, the application API will respond with the secure customer records.
{ "patient_id": "12345", "name": "John Doe", "age": 89, "sale_type": "cash", "sales_invoice": ["Service A", "Item/Product B"] }
This process ensures that only authorized applications can access sensitive customer information, complying with THE DATA PROTECTION ACT
Ready to Get Set Up?.
Contact us today:
Mobile Number: +254 790 360 360 or +254 711 082 360
Email: [email protected]
Slade360 eTIMS APIs are developed using industry best practices and the highest security standards
Updated 4 months ago